How to Convert a String to Unicode "\u0XXX" Symbols, but Only the Special Characters, using JavaScript
By AstroMacGuffin dated Mon Jul 25 2022 14:31:51 GMT+0000 (Coordinated Universal Time) last updated Sun Jul 31 2022 19:41:51 GMT+0000 (Coordinated Universal Time)The problem: You have a string that needs to be converted to Unicode symbols, *but only the special characters.* You want to leave the spaces and punctuation marks alone. Solution within!
This was a problem that came up in the JavaScript Mastery discord server, and I wasn't giving up until I figured it out.
```js
let thestring = 'A string with a β';
for (let x = 0; x < thestring.length; x++) {
if (thestring.charCodeAt(x) > 127) {
let before = thestring.substring(0, x);
let problem = thestring.substring(x, x+1);
let after = thestring.substring(x+1);
problem = problem.codePointAt(0);
problem = '\\u0' + problem.toString(16);
thestring = before + problem + after;
}
}
console.log(thestring);
```
Let's break that down:
```js
let thestring = 'A string with a β';
```
`β` is our Unicode character we want to convert into a \u0XXX value.
```js
for (let x = 0; x < thestring.length; x++) {
```
We're going to loop through the characters of `thestring`...
```js
if (thestring.charCodeAt(x) > 127) {
```
...and check their character codes, one by one. The first 128 character codes are ASCII symbols. Everything else is Unicode.
```js
let before = thestring.substring(0, x);
let problem = thestring.substring(x, x+1);
let after = thestring.substring(x+1);
```
Using `thestring.substring()` we've now broken the string down into what comes before and after the problem, and the single-character `problem` itself.
```js
problem = problem.codePointAt(0);
```
This gets the Unicode value of the character at position 0 of the `problem` variable.
```js
problem = '\\u0' + problem.toString(16);
```
`problem.toString(16)` converts the code point integer to a hexadecimal string, which we prepend with `\u0` to make it a Unicode symbol.
```js
thestring = before + problem + after;
```
We put the string back together and continue the loop.
```js
}
}
console.log(thestring);
```
Try it yourself!
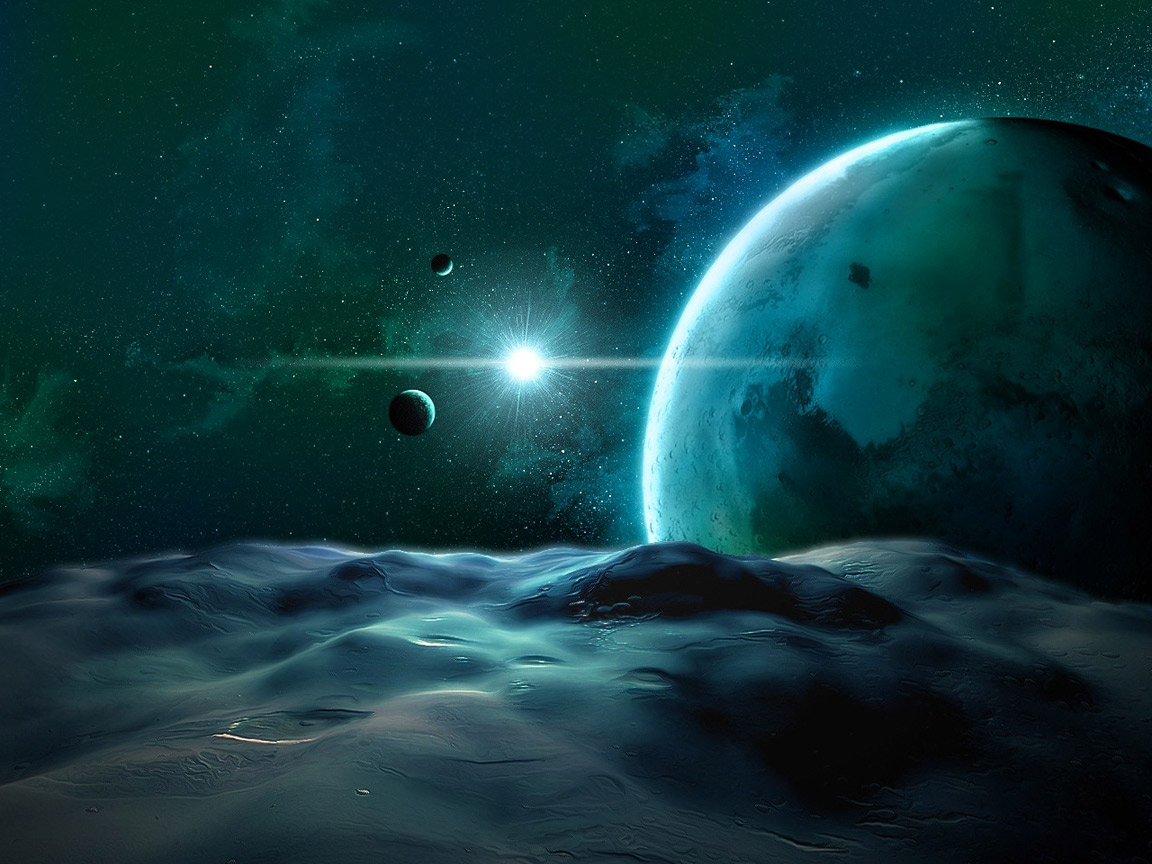
About the author of this piece, AstroMacGuffin: "I enjoy coding in Node.js and JavaScript as a hobby. I find Node.js to be a welcome escape, after a lifetime of code, with previous experience with PHP and JavaScript on the LAMP stack. I'm one of those people who spends way too much time herding electric sheep in front of a computer. You can find me on the JavaScript Mastery discord, where I'm a moderator, or on the Classic Shadowrun discord, where I'm the owner and founder. "