How to Code: The Fundamentals, pt 0
By AstroMacGuffin dated Sat Aug 06 2022 15:16:25 GMT+0000 (Coordinated Universal Time) last updated Sat Aug 20 2022 17:52:38 GMT+0000 (Coordinated Universal Time)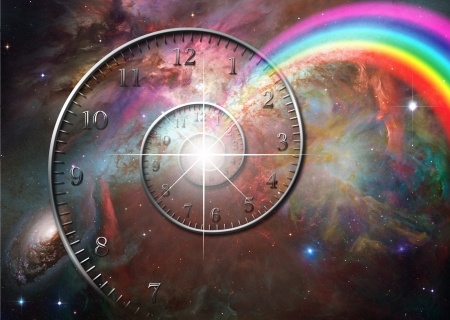 With parts 1 and 2 of this series already out, I now realize I skipped a few topics crucial to many students. Things like:
- how to run JavaScript code,
- how to find bugs in JavaScript code,
- what is Node.js,
- what's the difference between Node.js and JavaScript,
- what editor should I use to code in JavaScript,
- what is HTML,
- what is DOM,
- what is an NPM package,
- etc
This post is for beginners, and those whose experience mainly consists of jumping in too deep with not enough fundamental knowledge. Let's get you sorted on the bare beginnings of how to code in JavaScript.
### About this Lesson
Someone's got to teach you the bare-bones basics of the JavaScript world. Today's lesson is a step back to make sure you don't get left behind at the starting line.
### How to Run JavaScript Code
There are two main ways to run JavaScript code: in the web browser, or in a command terminal via something like Node.js. A web browser is very different from a command terminal, so let's look at how to do each of these two. Then comes a few key reasons why front-end JavaScript differs from Node.js JavaScript.
#### Running JavaScript Directly in the Browser
We normally load a web page either by typing its address, or its title if we've been there before, or some kind of search. But you can also load a web page directly from files on your computer. With Ctrl-O, the browser can open web pages we've created in a text editor. Try saving this as `test.html`:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<title>Starter Web Page</title>
<script>
// put your JavaScript code below this line
// put your JavaScript code above this line
</script>
</head>
<body>
<!-- Put your HTML code below this line -->
<!-- Put your HTML code above this line -->
</body>
</html>
```
It's as simple as that. Put some JavaScript code in where the code comments say to put them:
```html
<script>
// put your JavaScript code below this line
// put your JavaScript code above this line
</script>
```
For example:
```html
<script>
// put your JavaScript code below this line
alert('Hello, World!');
// put your JavaScript code above this line
</script>
```
Now to save that and load it... but how do you do that? If you're clear on saving a plaintext file as .html and loading it in the browser, you can skip this checklist:
1. First, scroll up 2 examples to the full web page sample, the one that ends with <`/html`>. Position your mouse cursor to the right of <`/html`> at the bottom. Hold down the left mouse button and move your mouse until the cursor is to the left of <`!DOCTYPE html`>. This should highlight all the code and only the code.
2. Next, hold down Ctrl on your keyboard, and press C (and then release both). You've now copied the code.
3. Next: Windows users should find Notepad in the Start Menu or Cortana search. Mac users should find TextEdit. Linux users, you probably know what you're doing, but if you don't, you probably want to run gEdit or Kate.
4. Once you have your plaintext editor in front of you, hold down Ctrl and press V (and then release both). This should paste the code into the text editor.
5. Hold down Ctrl and press S (the usual notation for that instruction would be Ctrl-S, by the way). A dialog box should appear offering the chance to Save but it will also have a somewhat subtle button to create a New Folder.
6. I recommend navigating in the Save pop-up to `Documents` or your equivalent home documents folder, and then creating a `src` folder. (You'll be creating a lot of source files from now on, and they need somewhere to go.)
7. Then double-click the new "src" folder.
8. If you're in Windows, make sure "Save as type" is set to "All Files". Then (on whatever platform) name the file something like `template-code-blank.html` and click the Save button. Now you can copy-paste this file whenever you need a blank HTML file with a `script` tag.
9. Next: go back to your web browser. Press Ctrl-O. Navigate to the `src` subfolder in your home documents folder, or wherever you just saved the file. Double-click on the desired `.html` file or click on it once and then click "Open". The browser should now be displaying your custom HTML file.
10. Make changes in the text editor, save them in the text editor, and then refresh the web browser by pressing the F5 key (top row of the keyboard, probably above the 5).
If you went with my suggested change, by adding this:
```js
alert('Hello, World!');
```
...a pop-up should appear saying simply "Hello, World!".
Welcome to the world of client-side* JavaScript!
*A word about naming. Browser-side JavaScript is often called "client-side code". (This is because the browser is a client app in a client/server relationship with various web servers, usually over the network.)
Crucial Links: [Absolute Beginner Tutorial for JavaScript](https://www.w3schools.com/js/DEFAULT.asp) | [Slightly More Advanced JavaScript Tutorials](https://developer.mozilla.org/en-US/docs/Web/javascript#tutorials)
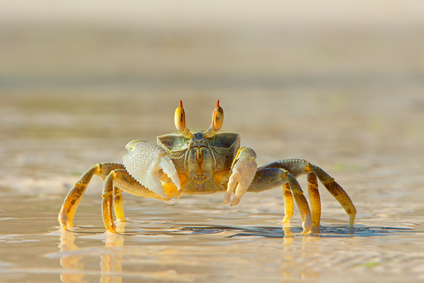
#### Running JavaScript via Node.js
And then there is server-side JavaScript. **I strongly recommend** you get comfortable with both client-side and server-side JavaScript, and with the differences between them, before you even think about job-hunting in this field. I will assume a higher experience level with computer use generally, when discussing Node. If instructions like "run the installer you just downloaded" aren't immediately comfortable for you, I recommend learning more about basic computer use before trying to learn Node.js again. Node is extremely powerful -- a programmer can have big oopsie poopsie, getting it wrong with Node.
Running Node code starts with [downloading and installing Node.js](https://nodejs.dev/download/). Download and run the installer. It will modify your path so that `node` and `npm` can be run conveniently on the command line.
First create a new folder for the project, `cd` into that folder, and type `npm init` to create a new project. There will be a few prompts. Remember the filename you choose for your main script. When `npm init` finishes, create a file with the same filename as your main script. Put your JavaScript code in that file. Then, back to the command line, you simply type `node .` (with a space before the dot). Assuming your command line terminal is still in the same folder as the script (which should be the same folder as where you ran `npm init`), your script will run.
Crucial Links: [Node manual quick-reference](https://manpages.org/nodejs) | [NPM manual quick-reference](https://manpages.org/npm)
### What is Node.js?
Node.js is the most popular flavor of "server-side JavaScript". Node code doesn't run in the browser. Instead there's a special program, `node.exe`, that runs the code outside of the web browser, usually in a command line terminal. As a server-side technology, Node is most commonly used as an alternative to PHP, ASP, JSP, Python, Perl, and other server-side languages.
Because server-side technologies are very powerful, I always recommend a strong foundation in general computer knowledge and browser-side JavaScript before diving into Node.
Crucial Links: [Nodejs.dev Download](https://nodejs.dev/download) | [Nodejs.dev Documentation](https://nodejs.org/en/docs/)
### What is an NPM Package?
NPM is the **N**ode **P**ackage **M**anager, but don't get into any bar fights defending that because actually I lied, it's called something totally other than **N**ode **P**ackage **M**anager. The name I came up with just makes more sense and helps people remember the command. NPM is a convenient way to find and install add-on packages for Node. Think of NPM packages like Minecraft mods... some of them do just one little feature, while others comprehensively change the game. For example, you could create a web server using Node's built-in `http` and `https` modules, but why would you ever do that when the NPM package `express` exists? (Actually, bad example, since I'm using both Express and the built-in stuff.) Why would you start over from raw network code and Discord's API documentation when you can just `npm install discord.js`, wait a few seconds while NPM works that crazy science, and then get on with coding your bot?
So when you're trying to find out if there's a package to take some of the load off your project, point your favorite web search at "NPM (whatever feature)" for a good time. But here's a tip, you'll need the right search-fu. Getting what you want out of the NPM package library is a matter of remembering: you're not likely to find the whole car, but you are likely to find the tools and the parts.
NPM is free, comes prepackaged with Node.js, and there is no signup process to access the packages. As far as I'm aware, all the packages themselves are free, although many of them (rightly) seek crowd funding on an ongoing basis. Subtly. You won't see ads in your apps.
### What's the Difference between Node.js and JavaScript?
You can't run browser-side JavaScript in Node.js, and you can't run Node code in the browser. That's difference #1.
The language you use to include modules is different. Also, you don't have access to the DOM in Node.js unless you install a package, and even then it's only for text parsing -- there's no way in Node.js to directly act upon elements in a browser window, like you can with browser-side JavaScript.
You can't make a web server with browser-side JavaScript, nor any kind of server -- that requires Node. You will one day find an NPM package you really really want to use in a browser-side JavaScript project, but you won't be able to: NPM is the ***Node*** Package Manager*, and its packages only work in Node.js projects.
*That's not actually what NPM stands for, but the History of Programmer Culture is a whole separate classroom, and we don't have time in this class to go into why programmers love recursive, self-referential acronyms so much. Moving on...
Despite those differences, Node and JavaScript have much more in common than not. Node *is* JavaScript, just done differently. Experience you gain working with either flavor of JavaScript will translate almost 100% to the other flavor of JavaScript.
### How to Find Bugs in JavaScript Code
The most common tool you'll ever use for debugging your code is a simple call to `console.log()`. The `console.log()` method outputs a message to the console -- in the browser, that means the Developer Tools console; in Node.js, it means the command line terminal where you launched the `node` program.
So how is `console.log()` useful for bug hunting? You output your variables to make sure they contain the values you expect them to contain. You output simple markers to make sure control flow is doing what you expect it should do, and to make sure asynchronous functions aren't finishing after you expect them to.
Another powerful tool is the `try` / `catch` block. The `try` statement begins a special code block / control flow structure. What's special about it? If anything throws an error within the `try` block, the commands after it (in that `try` block) will be skipped, and control flow skips to the `catch` block. But what's even better is that this technique allows you to catch errors (literally `catch` them) that might not have been reported to you otherwise. Here's how you use `try` / `catch`:
```js
try {
// code goes here
// if any line here fails, the rest of the try block will be skipped
}
catch (error) {
// control flow goes here if there's any error in the try block
console.log(error);
}
```
I can't overemphasize the importance of `try` / `catch` blocks because of the hidden errors they reveal.
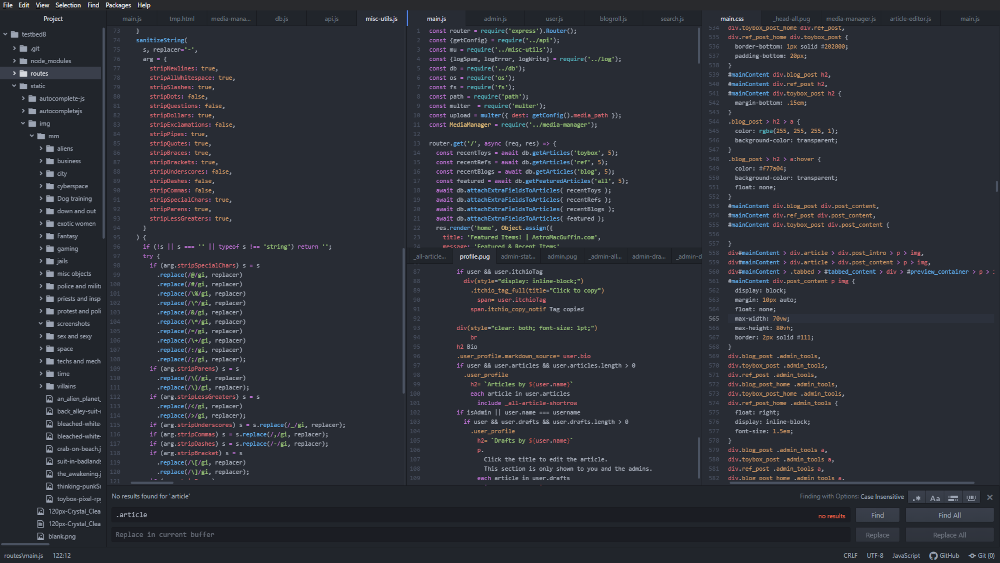
### What Editor Should I Use to Code in JavaScript?
There are two leading answers to this question, and I'll try to present the options without bias. For the record, I use Atom.
- VS Code: Much more newbie-friendly; for Node projects, VS Code has an integrated command line console so you don't need to Alt-Tab back and forth between coding and testing; for browser-side projects, VS Code has a convenient button for launching your changes in a browser window; live syntax checking attempts to find your mistakes before you run them.
- Atom: Much more minimal interface, giving you more space for code; Atom is hackable, with a built-in package manager and a vibrant community who make add-on packages for the editor -- you can add new features via point, click, and search; very fast, the interface doesn't slow you down from loading any resource-heavy bells and whistles.
I realize I probably just convinced most of you students to use VS Code, and that's fine, it's the obvious choice for newbie programmers. But just remember Atom has everything you actually need, so if you ever outgrow VS Code, Atom will still be there.
Crucial Links: [Atom homepage](https://atom.io) | [VS Code homepage](https://code.visualstudio.com/Download)
### What is HTML?
HTML is the language we use to make web pages. HTML stands for **H**yper**T**ext **M**arkup **L**anguage. Although there are frameworks that seem to make web pages without HTML, in the end those frameworks all output HTML to the browser: that includes React, Angular, Vue, etc. It's best to think of an HTML document as a bunch of nested block-level elements. Here's what I mean by that:
```html
<!doctype html>
<html>
<head>
<title>My First Web Page!</title>
<head>
<body>
<h1>It's a Web Page!</h1>
<p>I can't tell you how <strong>happy</strong> I am to have made it!</p>
</body>
</html>
```
Notice that the second line says <`html`> and the last line says <`/html`>? That's a block-level element, made of an opening tag and a closing tag. Nested within that element are two other block-level elements, the <`head`> and the <`body`>. The actual web page goes in the <`body`> block, as you can see from the <`h1`> block (level 1 header) and the <`p`> block (paragraph). Nested inside the paragraph is a <`strong`> block, which is HTML for **bold text**. With very few exceptions, everything is structured this way in HTML -- as an opening and closing tag, with possibly text and/or other tags between. If you go on to create web apps, it's highly recommended you learn a templating language as quickly as possible. **Pug** for example is a very convenient way to make templates that are similar to HTML but much quicker to work with.
Crucial Links: [Absolute Beginner's Tutorial on HTML](https://www.w3schools.com/html/html_intro.asp) | [HTML Tutorials & References](https://developer.mozilla.org/en-US/docs/Web/HTML)
### What is CSS?
CSS stands for **C**ascading **S**tyle **S**heets. CSS is a language for defining the style of a web page. I'm not going to lie to you: CSS is very much a thing that takes 15 minutes to learn and a lifetime to master. The basic structure of a CSS file is that you have selectors and rules. A selector is how you identify what part of the web page the rule applies to. The rule is a stack of CSS attributes and values. For example:
```css
h1 {
font-style: italic;
}
p strong {
font-size: 18pt;
color: #550000;
}
h1, p strong {
font-weight: bolder;
}
#adjective {
color: #0a3154;
}
```
We have four rules in the CSS code above.
- The first rule has one selector: every <`h1`> tag.
- The second rule has one selector: every <`strong`> tag that's nested in a <`p`> tag.
- The third rule has two selectors, separated by a comma: every <`h1`>, and every <`strong`> tag that's nested inside a <`p`> tag.
- The fourth rule has one selector: whatever tag has the id, `adjective`. (Note, ID's should always be unique on a page.)
There's a conflict in these CSS rules: two different colors are being applied to the same <`strong`> element. One color is being applied because the <`strong`> element is nested within a <`p`> tag, the other color is being applied because the <`strong`> tag has the `id="adjective"` attribute. The browser decides which CSS selector is more specific, and applies that one. Since `#adjective` is more specific than `p strong`, we get our adjective in blue instead of red.
There's been a glut of new CSS features over the past decade. But it's all easy to find with a web search, for example "CSS bold text", or "CSS change font", etc. It's worth taking time to browse through an overview of all the features, just so you can know what's there. You'll be searching for specific answers on CSS attributes and their allowable values and how each value behaves, for the rest of your natural life. There's no way to memorize this many details.
Crucial Links: [A CSS Tutorial for Absolute Beginners](https://www.w3schools.com/Css/css_intro.asp) | [Tutorials & References for Everyone](https://developer.mozilla.org/en-US/docs/Web/CSS)
### What is DOM?
When HTML goes into the browser, the browser turns that HTML into DOM. DOM stands for **D**ocument **O**bject **M**odel. All those block-level elements I explained above, in the "What is HTML" section, become JavaScript objects thanks to DOM. Every attribute and style, whether explicitly defined or computed, can be accessed and usually changed from JavaScript thanks to DOM. This is how web sites do animation, such as having the content swoosh onto the screen or fade away. It's also a huge part of how you achieve a website that only has one constantly-evolving "page" such as GMail or Facebook.
But enough talk, let's show our code with a real example:
`The HTML:`
```html
<!doctype html>
<html>
<head>
<title>My First Web Page!</title>
<head>
<body>
<h1>It's a Web Page!</h1>
<p>I can't tell you how <strong id="adjective">happy</strong> I am to have made it!</p>
</body>
</html>
```
I've copy-pasted the above example from the question "What is HTML" and made one tiny adjustment. The bold text block signified by the <`strong`> tag now has an attribute defined. It's the `id` attribute and I've set it to the value `adjective`. Now let's change the wording of this web page using JavaScript:
```js
// get the strong node as a DOM object in a variable
let adj = document.getElementById('adjective');
// change the text in the strong node
adj.innerHTML = 'EXCITED';
```
It's as simple as that. Dynamically creating new elements isn't much harder, but you get the idea.
Crucial Links: [A Beginner's Guide to DOM](https://www.w3schools.com/js/js_htmldom.asp) | [Your "Forever" Guide to DOM](https://developer.mozilla.org/en-US/docs/Web/API/Document_Object_Model)
### Lesson Conclusion
In this lesson we've seen brief primers to all the following technologies: two flavors of JavaScript, plus CSS, HTML, DOM, and NPM. And, we got hands on with most of it, to show it's really not intimidating. There's just a lot of little steps to everything. We've learned how to use `console.log()` and where to find its output, and we've also learned how to use it effectively as a programmer's troubleshooting tool. We learned about the `try` / `catch` block that I can't recommend highly enough. And, I covered the usual reasons why people either choose VS Code or Atom as their code editor of choice.
With Lesson 0 out of the way, I hope you're now prepared for [part 1 of this series, "How to Code: Fundamentals"](https://astromacguffin.com/ref/id/62ebac50209885383e63d856).
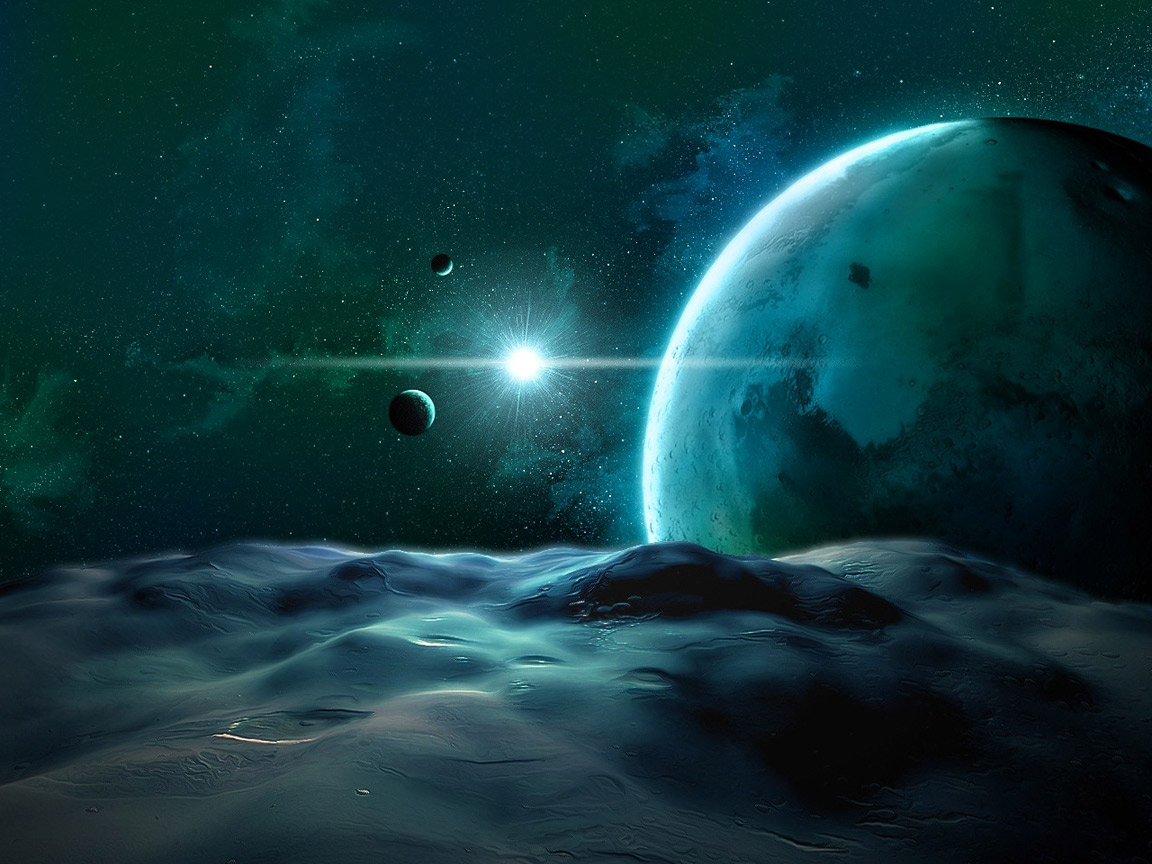
About the author of this piece, AstroMacGuffin: "I enjoy coding in Node.js and JavaScript as a hobby. I find Node.js to be a welcome escape, after a lifetime of code, with previous experience with PHP and JavaScript on the LAMP stack. I'm one of those people who spends way too much time herding electric sheep in front of a computer. You can find me on the JavaScript Mastery discord, where I'm a moderator, or on the Classic Shadowrun discord, where I'm the owner and founder. "